I’m still looking for a new project to work on. Previously, I had mentioned that I built a Podcast Downloader. That project will (still) likely never see the light of day.
I’ve also been working on something else. This is a replica of Apple’s Stopwatch functionality in the Clock app. This project has been open-sourced on my Github account. You can view the project here.
It’s not a complete replica of the stopwatch functionality. I only implemented the digital version and not the analog version. I’m not sure I have the patience to attempt to implement the analog version. I also didn’t implement the iPad or macOS versions of the stopwatch. I only focused on the iPhone version.
Why?
Why did I do this? I did this so I can continue to improve as a software engineer.
I don’t get as much time to write code anymore. At least not as much as I want to. Even at my day job, I spend more time not writing code. I like to believe that projects like this keep me from getting rusty.
As I’ve said, I don’t currently have a personal project to work on. So something like this helps me to continue to learn new technologies and stay current.
Development Process
This project was developed over roughly two weeks. I only worked on it for roughly an hour a night and not every night.
The project has been sitting untouched for a few weeks on my laptop. I wasn’t sure if I was going to post it at all. I finally decided to post it.
The project wasn’t code reviewed or tested too rigorously for issues. It probably isn’t my best work. It also isn’t my worst work. Overall, I’m happy with how it turned out.
Technologies
The project is written in Swift, SwiftUI, and Combine and uses an MVVM-like architecture. I am still learning each of these technologies and approaches.
Swift
I’ve been using Swift since it was announced at WWDC in 2014. I haven’t written much in Objective-C in a few years. Swift is the way to go.
Even though I have been using Swift for years, I still have a lot to learn. I didn’t learn anything new with Swift in this project. Instead, I focused on learning more about the next two technologies.
SwiftUI
I’m still new to SwiftUI. I’ve used it here and there in the past. However, I’ve never built an entire app using SwiftUI. This is a first for me.
This app is simple, but I’ve learned valuable lessons from what I have done.
At work, we’ve been slowly writing new features using SwiftUI. I think the code I wrote here will help at work.
Combine
I’ve been interested in Combine since it was announced. At the time, I had started using Combine for simple things, like dealing with notifications from NotificationCenter.
We use Combine (and RxSwift) heavily at work. It took me a while to start learning the concepts. I still need to occasionally look at guides on Combine. Any extra experience I can get with Combine (and reactive programming) is welcome to me.
I’ve come a long way with my Combine knowledge in the last few years. But I have so much more to learn.
Testing
I have always been an advocate for unit testing. The problem is that unit testing is always the last on my mind when developing. I love the idea of having unit tests, I’m just not very dedicated to writing them.
I’m trying to do a better job of that, including this project. I probably don’t need any unit tests for something like this. The entire project is an experiment. But I need to start somewhere. So, I included unit testing.
I could improve the unit testing in many ways. I could probably use something like ViewInspector to test the SwiftUI views.
I should also provide a deterministic way to test the display strings that are generated by the StopwatchViewModel
class while the timer is running. Instead of hard-coding the .main
RunLoop in this method:
private func startTimer() {
timerCancellable = Timer
.publish(every: 0.01, on: .main, in: .common)
.autoconnect()
.sink { [self] newDate in
let difference = (date ?? .now).distance(to: newDate)
date = newDate
overallInterval += difference
currentLapInterval += difference
}
}
I could start the timer, stop it, and ensure the value is not the default display string value of “00:00.00”, but that’s not a great test. Ideally, I would advance the timer at different intervals and test the results.
I wonder if Point-Free’s Combine Schedulers Publishers.Timer would work here. This approach would allow using any scheduler (including their TestScheduler) instead of RunLoop
. This would allow me to advance the schedule as I desired and make the tests more deterministic.
I didn’t use this approach only because I didn’t want to depend on external libraries for this project. It was initially a thought experiment. If I would ever want to make this a full-scale app, I would investigate using Publishers.Timer
.
Coverage
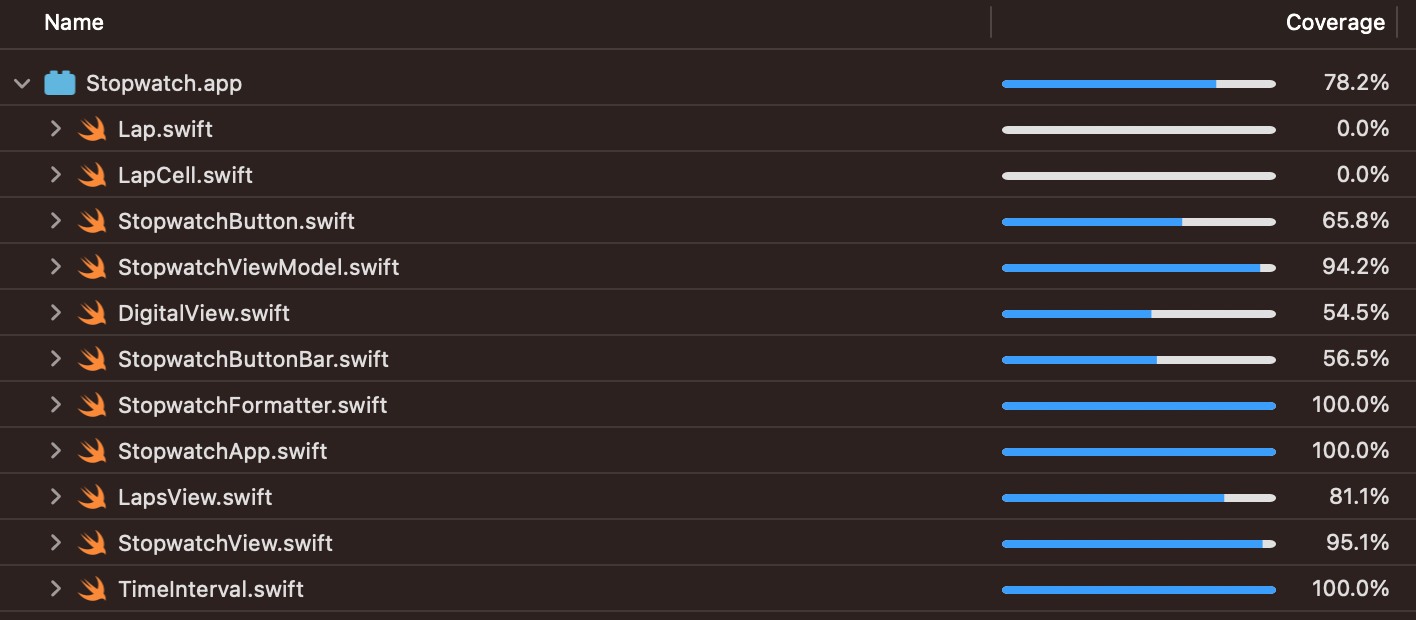
The coverage on the app is decent. It’s at about 78%. It could be better. I think if I were using a testing framework like ViewInspector, I could test the SwiftUI views and get better coverage.
Summary
I’m happy with how this turned out. I didn’t spend a crazy amount of time on it. I don’t have a lot of time each night to write code. Often, I don’t get any time at night to write code.
This was a fun little experiment.